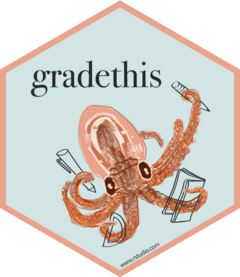
Signal a failing grade if mistakes are detected in the submitted code
Source:R/graded.R
fail_if_code_feedback.Rd
fail_if_code_feedback()
uses code_feedback()
to detect if there are
differences between the user's submitted code and the solution code (if
available). If the exercise does not have an associated solution, or if there
are no detected differences between the user's and the solution code, no
grade is returned.
See graded()
for more information on gradethis grade-signaling
functions.
Usage
fail_if_code_feedback(
message = NULL,
user_code = .user_code,
solution_code = .solution_code_all,
...,
env = parent.frame(),
hint = TRUE,
encourage = getOption("gradethis.fail.encourage", FALSE),
allow_partial_matching = getOption("gradethis.allow_partial_matching", TRUE)
)
Arguments
- message
A character string of the message to be displayed. In all grading helper functions other than
graded()
,message
is a template string that will be processed withglue::glue()
.- user_code, solution_code
String containing user or solution code. By default, when used in
grade_this()
, .user_code is retrieved for the .user_code.solution_code
may also be a list containing multiple solution variations, so by default ingrade_this()
.solution_code_all is found and used forsolution_code
. You may also use.solution_code
if there is only one solution.- ...
Arguments passed on to
graded
correct
A logical value of whether or not the checked code is correct.
type,location
The
type
andlocation
of the feedback object provided to learnr. See https://rstudio.github.io/learnr/exercises.html#Custom_checking for more details.type
may be one of "auto", "success", "info", "warning", "error", or "custom".location
may be one of "append", "prepend", or "replace".
- env
Environment used to standardize formals of the user and solution code. Defaults to retrieving .envir_result and .envir_solution from
parent.frame()
.- hint
Include a code feedback hint with the failing message? This argument only applies to
fail()
andfail_if_equal()
and the message is added using the default options ofgive_code_feedback()
andmaybe_code_feedback()
. The default value ofhint
can be set usinggradethis_setup()
or thegradethis.fail.hint
option.- encourage
Include a random encouraging phrase with
random_encouragement()
? The default value ofencourage
can be set usinggradethis_setup()
or thegradethis.fail.encourage
option.- allow_partial_matching
A logical. If
FALSE
, the partial matching of argument names is not allowed and e.g.runif(1, mi = 0)
will return a message indicating that the full formal namemin
should be used. The default is set via thegradethis.allow_partial_matching
option, or bygradethis_setup()
.
Value
Signals an incorrect grade with feedback if there are differences between the submitted user code and the solution code. If solution code is not available, no grade is returned.
See also
Other grading helper functions: graded()
, pass()
, fail()
,
pass_if()
, fail_if()
, pass_if_equal()
, fail_if_equal()
.
Examples
# Suppose the exercise prompt is to generate 5 random numbers, sampled from
# a uniform distribution between 0 and 1. In this exercise, you know that
# you shouldn't have values outside of the range of 0 or 1, but you'll
# otherwise need to check the submitted code to know that the student has
# chosen the correct sampling function.
grader <-
# ```{r example-check}
grade_this({
fail_if(length(.result) != 5, "I expected 5 numbers.")
fail_if(
any(.result < 0 | .result > 1),
"I expected all numbers to be between 0 and 1."
)
# Specific checks passed, but now we want to check the code.
fail_if_code_feedback()
# All good!
pass()
})
# ```
.solution_code <- "
# ```{r example-check}
runif(5)
# ```
"
# Not 5 numbers...
grader(mock_this_exercise(runif(1), !!.solution_code))
#> <gradethis_graded: [Incorrect] I expected 5 numbers.>
# Not within [0, 1]...
grader(mock_this_exercise(rnorm(5), !!.solution_code))
#> <gradethis_graded: [Incorrect]
#> I expected all numbers to be between 0 and 1.
#> >
# Passes specific checks, but hard to tell so check the code...
grader(mock_this_exercise(runif(5, 0.25, 0.75), !!.solution_code))
#> <gradethis_graded: [Incorrect]
#> In `runif(5, 0.25, 0.75)`, I expected `0` where you wrote
#> `0.25`.
#> >
grader(mock_this_exercise(rbinom(5, 1, 0.5), !!.solution_code))
#> <gradethis_graded: [Incorrect]
#> I expected you to call `runif()` where you called `rbinom()`.
#> >
# Perfect!
grader(mock_this_exercise(runif(n = 5), !!.solution_code))
#> <gradethis_graded: [Correct]
#> Someone knows what they're doing :) Correct!
#> >